Setup Instructions
Place the following code where you'd like Veedi player to load:
<script type="text/javascript" id="veediInit">
var _v,settings = {
game : "var_GAMENAME", // Game name (Variable)
publisherId : 12345, // Publisher ID (provided by our side)
onVideoFound : function() { // perform an action if video found
},
onVideoNotFound : function() { // perform an action if video NOT found
},
width : 700 // Veedi player width
};
(function(settings) {
var vScript = document.createElement('script');
vScript.type = 'text/javascript'; vScript.async = true;
vScript.src = '//www.veedi.com/player/embed/veediEmbed.js';
vScript.onload = function(){_v = new VeediEmbed(settings);};
var veedi = document.getElementById('veediInit'); veedi.parentNode.insertBefore(vScript, veedi);
})(settings);
</script>
If your site is using HTTPS protocol make sure you use this link vScript.src = '//www.veedi.com/player/embed/veediEmbed.js' without HTTP protocol.
"game"
Replace "var_GAMENAME" with the game name you would like to get video for.
Please note: The game name should be in English only.
If you are a global site please make sure you pass English game name in this parameter.
Examples for valid game names:
Fireboy & Watergirl 4: Crystal Temple, Red Ball 4 Vol. 3, Papa's Burgeria, L.A. REX, RACHEL'S KITCHEN GRANDPRIX: CAKE, etc…
"publisherId"
Your publisher ID (ask your publisher id from your contact at Veedi).
"onVideoFound" (highly recommended)
Pass to this field the function you want to be called if the video found and the player appears.
- Usage example:
You can draw special section for the player on the page - with this call back you can trigger this section not to draw itself when not-needed (when a video is not found).
"onVideoNotFound" (highly recommended)
Pass to this property the function you want to be called if the game name provided in the "game" property is not found and the player will not appear.
"width"
Define the width of the player.
* Height – will be generated automatically to keep the correct aspect ratio (16:9).
"Ads.txt"
Please add the below line to your Ads.txt file.
google.com, pub-5647035702750713, DIRECT, f08c47fec0942fa0
google.com, pub-7347882540213117, DIRECT, f08c47fec0942fa0
Advanced implementation (recommended):
1. Callback functions
2. Localization
3. Mobile web implemenation
4. API
1. Callback functions
<script type="text/javascript" id="veediInit">
var _v,settings = {
game : "var_GAMENAME",
publisherId : 12345,
onVideoFound : function() { },
width : 700,
// callback for logging error types and info
onError : function(data) { YOUR CODE },
// callback for knowing when player toggles full screen mode
onFullscreen : function(boolean) { YOUR CODE }
};
(function(settings) {
var vScript = document.createElement('script');
vScript.type = 'text/javascript'; vScript.async = true;
vScript.src = 'http://www.veedi.com/player/embed/veediEmbed.js';
vScript.onload = function(){_v = new VeediEmbed(settings);};
var veedi = document.getElementById('veediInit'); veedi.parentNode.insertBefore(vScript, veedi);
})(settings);
</script>
"onError"
Pass function that will receive error logs from the player when implementation problem occurred.
- Usage example:
You can console.log data received from this callback and see clarification regarding specific error type in browser's console.
"onFullScreen"
Fires every time when user toggles a full screen mode of the player. Function will receive true/false state of full screen (true – full screen enabled, false – full screen disabled).
- Usage example:
Useful for triggering page behavior when entering browser full screen mode.
2. Localization
Veedi player supports localization features:
<script type="text/javascript" id="veediInit">
var _v,settings = {
game : "var_GAMENAME",
publisherId : 12345,
width : 700,
// Define UI language of the player (ISO 639-1) elements
lang : "en",
// Define special naming title – for translated titles
gametitle : "Your defined title to the game"
};
(function(settings) {
var vScript = document.createElement('script');
vScript.type = 'text/javascript'; vScript.async = true;
vScript.src = 'http://www.veedi.com/player/embed/veediEmbed.js';
vScript.onload = function(){_v = new VeediEmbed(settings);};
var veedi = document.getElementById('veediInit'); veedi.parentNode.insertBefore(vScript, veedi);
})(settings);
</script>
"lang"
Determine the language of player's UI elements (instead of English) - just pass the 2 letter (ISO 639-1) language code.
- Usage example:
Input "de" for Deutsch language.
"gametitle"
This property would determine what title will be shown on player's head.
- Usage example:
Useful for cases of translated names of games.

3. Mobile web implemenation
Veedi unified embed code
Veedi embed code is a global code. The player itself will recognize mobile device and will load appropriate UI optimized for mobile view.
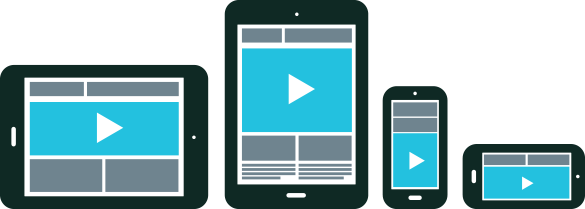
* Important note Since mobile expirence is responsive - in mobile mode the player would not keep auto 16:9 aspect ratio.
You need to make sure that the container of the player has both "height" and "width" properties defined in order for the embed code to understand in which dimensions to draw the player.
Other than that - everything is happening behind the scenes, no special attention from you is required :).
4. API
Veedi player supports some handy API functions:
These functions allow developer to control several states of the player without involving the user.
"play()"
Equivalent to “Play” button.
"pause()"
Equivalent to “Pause” button.
"mute()"
Mutes the video sound.
"unmute()"
Unmutes the player video (When, unmuting the player, it will change back to previous volume state, that was before video was muted).
"setVolume(20)"
You can specify Volume of the player by providing integer inside this function in range of 0-100. We advise to use multiplies of 10 such as 10, 20 and 30 etc…
"_v.enableWTbutton()"
If you want to trigger the walkthrough dynamic button by need - you can call it with this command.
How to use API?
In example below your Video will start playing in muted mode:
<script>
_v.mute();
</script>
- Usage example:
You can mute the player sound while user interacts with the game – and un-mute the player sound when user focused again on the video.